Usable
A UsableItem is any item that can be triggered by the Use ability. The UsableItem will determine common use functionality such as the rate that the item is used or if the item uses an animation event to indicate that it is done being used.
The UsableItemAction comes with two ActionModuleGroups.
- Trigger: Used to define when the ability switched from start, use and complete often with animation events.
- Usable: Invokes an action at any step of the action as well as add conditions to prevent or force the action to start or stop.
The Item Action were built with modularity and flexibility in mind. Everything related to the if, when and how an item is used can be defined as ActionModules.
ActionModuleGroups are generic objects that only accepts a certain type of ActionModule. The common ActionModuleGroups for all UsableItemActions are the Trigger and Usable groups.
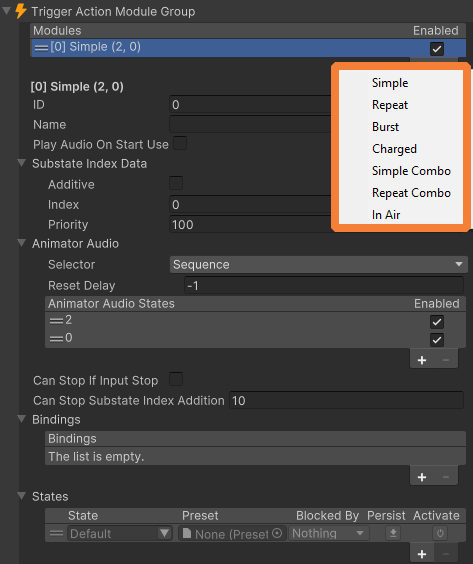

Trigger Modules
TriggerModules define how the UsableItem gets to start and stop. It usually uses an AnimatorAudioStateSet such that an animation can be played when triggered.
Simple
When the UseAbility starts the item use, the item is used once and then stops.
Repeat
The item continues to be used repeatedly at the use rate for as long as the use input is active.
Burst
The item will be used at a pre-defined set of times.
Charged
The item will be used once the use input is stopped. The charge time can be used to set a at a pre-defined set of times.
Simple Combo
Similar to Simple, but the use can chain after OnUseComplete rather than having to wait for the use stop. This module requires at least 2 states in the AnimatorAudioStateSet
Repeat Combo
Similar to Repeat, but the use can chain after OnUseComplete rather than having to wait for the use stop.
In Air
Similar to Simple, but can only be used while in air.
Usable Modules
The UsableModules are used to invoke function when starting, using, completing and/or stopping the item use. But it can do so much more.
Generic Item Effects
The Generic Item Effects module can run sub-effects when an item is used, completed or updated.
Active States
Activate or deactivate states while the item is equipped or being used.
Use Attribute
Use an attribute on the character when the item is used.
Character Use Attribute
Use an attribute on the item when the item is used.
Use Attribute Modifier Toggle
Used to modify an attribute when and/or while an item is used. It can be used to prevent the item from being used if the attribute is invalid. It also enables or disables a GameObjects depending if the item is being used or not.
Module State Switcher
Used for looping through a list of state options with always one selected.
Enable Can Start Use
Have a boolean to prevent the item from being used. It could be linked to a state or manually toggled in code.
Inspected Fields
In addition to the ModuleGroups the Usable Item Action does have its own fields.
Use Rate
The amount of time that must elapse before the item can be used again.
Face Target
Should the character rotate to face the target during use?
Stop Use Ability Delay
The amount of extra time it takes for the ability to stop after use. This is useful for preventing the item from immediately going back to the idle state after hip firing.
Use Event
Specifies if the item should wait for the OnAnimatorItemUse animation event or wait for the specified duration before being used. This field uses an Animation Event Trigger.
Use Complete Event
Specifies if the item should wait for the OnAnimatorItemUseComplete animation event or wait for the specified duration before completing the use. This field uses an Animation Event Trigger.
Force Root Motion Position
Does the item require root motion position during use?
Force Root Motion Rotation
Does the item require root motion rotation during use?