Impact Action Conditions
Impact Action Conditions allow you to determine when an impact happens based on a set of conditions. Conditions can be set on the source or the target of the impact. An example of an impact condition an object that can only be impacted by items from a certain category.
Conditional Impact Action
This impact action allows you to set a list of conditions and nest impact action in case the conditions pass or fails.
In the screenshot above if the condition succeeds it will deal the damage, if it fails it will play an audio clip. The RestDamageDataOnFail option can allow you to avoid propagating the damage if the condition is set before a simple damage within the same list.
Impact Condition Behavior
The impact condition behavior is a component that inherits ImpactConditionBehaviorBase and inherently the IImpactCondition interface. This component allows you to define a list of impact conditions on a GameObject. If this is paired with the CheckTargetImpactConditionBehaviour condition on the source it can allow the object that triggers the impact to follow the conditions set on the target.
An example usage of this is if you have a sword with a ConditionalImpactAction, in the list of conditions you have a CheckTargetImpactConditionBehaviour. On the target object you could then have a ImpactConditionBehaviour component with a CheckWeaponSourceDefinition condition similar to the screenshot below.
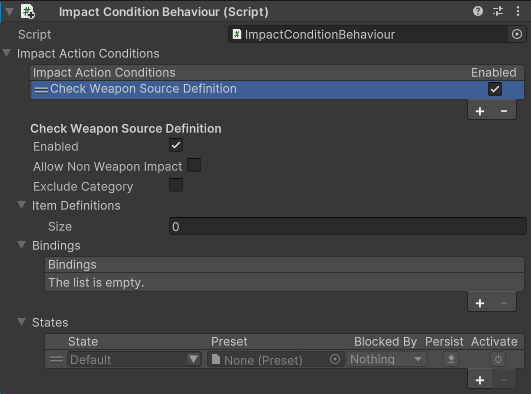
Conditional Impact Receiver
In some cases you may prefer to relay the Impact Action to the target. This way the target will choose what happens when impacted instead of the source checking for all possible conditions. In this case you must have an Impact Action that sends an impact event to the target. Similar to the ConditionImpactAction, Impact Actions can be set for both pass and fail
Additional ImpactCondition inheriting the IImpactCondition can be fetched automatically or set manually to add even more custom conditions.
Custom Impact Action Condition
Writing a custom Impact Action Condition is very simple. Simply inherit the “ImpactActionCondition” class and override the CanImpact function.
/// <summary> /// Checks the ObjectIdentifier on the target gameobject. /// </summary> [Serializable] public class MyImpactCondition : ImpactActionCondition { /// <summary> /// Internal, can the impact proceed from the context. /// </summary> /// <param name="ctx">Context about the hit.</param> /// <returns>True if the impact should proceed.</returns> protected override bool CanImpactInternal(ImpactCallbackContext ctx) { // Write your condition here, return true or false. } }
Existing Impact Action Condition
Here is a list of default Impact Action Conditions:
- CheckTargetImpactConditionBehaviour
- CheckWeaponSourceCategory
- CheckWeaponSourceDefinition
- ObjectIdentifierImpactCondition
- ProjectileImpactActionCondition