We're using the latest version of the Third Person Controller. With the Adventure view and movement type, the character moves forward when we strafe left or right. This is due to the character rotating in a sort of "arc" as can be seen in this GIF:
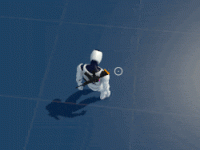
Changing the Motor Rotation Speed to 1 kind of fixes the issue but then the rotation of the character snaps immediately with no lerping so it looks pretty bad.
The RPG movement type doesn't seem to have this issue (if Previous Acceleration Influence is set to 0), but we are looking to use the Adventure type as it fits our game best.
This is a GIF from the Unity starter assets pack for their third person controller. Here you can see the character moves on the spot when hitting the strafe keys but still has a turning rotational lerp.
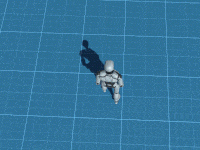
Any pointers on how to address this would be much appreciated.
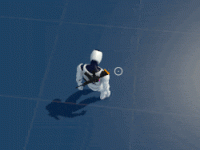
Changing the Motor Rotation Speed to 1 kind of fixes the issue but then the rotation of the character snaps immediately with no lerping so it looks pretty bad.
The RPG movement type doesn't seem to have this issue (if Previous Acceleration Influence is set to 0), but we are looking to use the Adventure type as it fits our game best.
This is a GIF from the Unity starter assets pack for their third person controller. Here you can see the character moves on the spot when hitting the strafe keys but still has a turning rotational lerp.
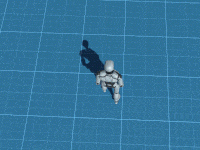
Any pointers on how to address this would be much appreciated.