I recently upgraded 1.2.15 to 1.2.18. The method CanAddItem in ItemSlotCollectionView now has another condition to check if the item is already in the collection.
I am adding a new item to the collection with this bit of code:
The ItemStack on the ItemInfo I create is null and so this new condition is crashing. I don't understand what the circled condition is checking and I think it might be wrong? Or, do I need to do some additional initialization on the ItemInfo before I try to add to the collection?
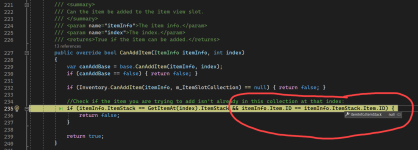
I changed this code as follows, because I think that is what the check should be?
I am adding a new item to the collection with this bit of code:
C#:
ItemInfo hotbarInfo = new ItemInfo(item, qty);
newItemInfo = HotBarSlots.AddItem(hotbarInfo, position);
The ItemStack on the ItemInfo I create is null and so this new condition is crashing. I don't understand what the circled condition is checking and I think it might be wrong? Or, do I need to do some additional initialization on the ItemInfo before I try to add to the collection?
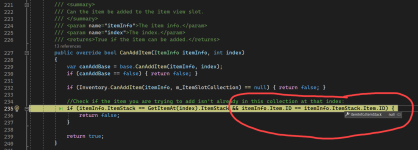
I changed this code as follows, because I think that is what the check should be?
C#:
//Check if the item you are trying to add isn't already in this collection at that index:
if (itemInfo.ItemStack != null && itemInfo.ItemStack == GetItemAt(index).ItemStack && itemInfo.Item.ID == GetItemAt(index).ItemStack.Item.ID) {
return false;
}