TPC 3.0.15
Hello, it seems feasible for Ability to inherit from BoundStateObject, and in my tests, it only requires a few code modifications to make it work successfully (although these code changes may potentially introduce some issues I haven't discovered yet, and it might have poor performance).
To successfully inherit Ability from BoundStateObject and display the Binding field in my test, there are three steps:
1. Modify the base class of Ability to be BoundStateObject.
2. Modify the base class of AbilityControlType to be BoundStateObjectControlType.
3. In the UltimateCharacterLocomotionInspector's ShowAbility method, pass the SerializedProperty of Ability to the FieldInspectorView.AddFields method.
After making these changes, you should be able to display Binding correctly, and you can modify and save it as well.
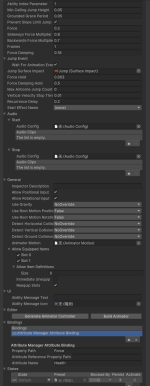
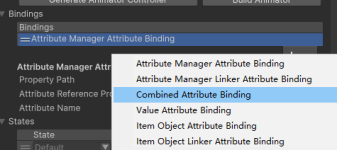
(These binding modules are ones I wrote)
Perhaps inheriting Ability from BoundStateObject is straightforward? I hope this modification method can help other developers, and I also hope that the official support for having Ability inherit from BoundStateObject will come sooner.
Hello, it seems feasible for Ability to inherit from BoundStateObject, and in my tests, it only requires a few code modifications to make it work successfully (although these code changes may potentially introduce some issues I haven't discovered yet, and it might have poor performance).
To successfully inherit Ability from BoundStateObject and display the Binding field in my test, there are three steps:
1. Modify the base class of Ability to be BoundStateObject.
Code:
[Serializable]
[UnityEngine.Scripting.Preserve]
public abstract class Ability : BoundStateObject
{
Code:
[ControlType(typeof(Ability))]
public class AbilityControlType : BoundStateObjectControlType
{
Code:
/// <summary>
/// Shows the selected ability.
/// </summary>
/// <param name="abilities">Should the abilities be shown? If false the item abilities will be shown.</param>
/// <param name="index">The index of the ability.</param>
/// <returns>True if the ability was shown.</returns>
private bool ShowAbility(bool abilities, int index)
{
var abilityContainer = (abilities ? m_SelectedAbilityContainer : m_SelectedItemAbilityContainer);
abilityContainer.Clear();
var ability = (abilities ? m_CharacterLocomotion.Abilities[index] : m_CharacterLocomotion.ItemAbilities[index]);
if (ability == null) {
return false;
}
SerializedObject serializedObject = new SerializedObject(m_CharacterLocomotion);
var abilitiesSerializedProperty = serializedObject?.FindProperty(abilities ? "m_Abilities" : "m_ItemAbilities");
var serializedProperty = abilitiesSerializedProperty?.GetArrayElementAtIndex(index);
var label = new Label(InspectorUtility.DisplayTypeName(ability.GetType(), true));
label.style.unityFontStyleAndWeight = UnityEngine.FontStyle.Bold;
abilityContainer.Add(label);
FieldInspectorView.AddFields(m_CharacterLocomotion, ability, Shared.Utility.MemberVisibility.Public, abilityContainer, (obj) =>
{
// The ability reference may have been updated.
if (abilities) {
m_CharacterLocomotion.Abilities[index] = obj as Ability;
} else {
m_CharacterLocomotion.ItemAbilities[index] = obj as ItemAbility;
}
Shared.Editor.Utility.EditorUtility.RecordUndoDirtyObject(target, "Change Value");
}, serializedProperty, null, true, null, false, null, this);
return true;
}
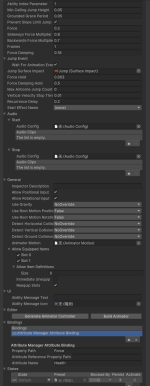
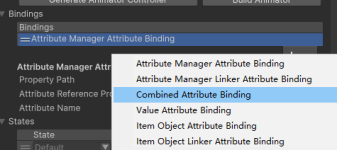
(These binding modules are ones I wrote)
Perhaps inheriting Ability from BoundStateObject is straightforward? I hope this modification method can help other developers, and I also hope that the official support for having Ability inherit from BoundStateObject will come sooner.