KrejwenBAN
Member
Hi,
I have a question about GenericObjectPool.
I am adding two resources by the following code:
Now I am trying to add notification about added resource by the following code:
In the first time I have the following values

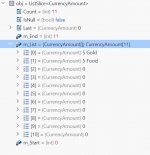
On second time (just add resource one more time) in the result I have
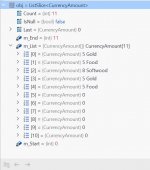
So data are incorrect. I think that the problem is in CurrencyCollection method which is not clear the data from pool before set it.
Can you tell me what can I do to fix this?
Inventory System version 1.1.7
I have a question about GenericObjectPool.
I am adding two resources by the following code:
C#:
public void AddResource(CurrencyCollection r, bool hideNotification = false)
{
_currencyOwner.AddCurrency(r);
_hideNotification = hideNotification;
}
Now I am trying to add notification about added resource by the following code:
C#:
private void CurrencyAmountOnOnAdd(ListSlice<CurrencyAmount> obj)
{
ResourceUpdate?.Invoke();
if (_hideNotification)
{
_hideNotification = false;
return;
}
if(obj.Count == 0)// || obj.Last.Amount == 0)
return;
_notificationSystem.Notify(new ResourceNotification(obj, false));
}
In the first time I have the following values

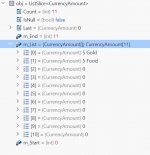
On second time (just add resource one more time) in the result I have
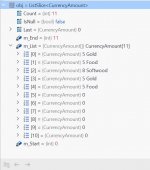
So data are incorrect. I think that the problem is in CurrencyCollection method which is not clear the data from pool before set it.
C#:
protected virtual bool AddCurrencyInternal(ListSlice<CurrencyAmount> currencyAmounts, double multiplier, bool notify)
{
var pooledArray = GenericObjectPool.Get<CurrencyAmount[]>();
var lhsCurrencyAmountCopy = ListSlice.CopyTo(m_CurrencyAmounts, ref pooledArray);
//m_CurrencyAmounts is about to be overwritten so return the array to the pool
GenericObjectPool.Return(m_CurrencyAmounts.Array);
var pooledArray2 = GenericObjectPool.Get<CurrencyAmount[]>();
var added = Addition(lhsCurrencyAmountCopy, 1d, currencyAmounts, multiplier, ref pooledArray2);
m_CurrencyAmounts.Initialize(pooledArray2, false, added.Count);
if (notify) {
var addedAmountPooledArray = GenericObjectPool.Get<CurrencyAmount[]>();
ConvertToDiscrete(currencyAmounts, multiplier, ref addedAmountPooledArray);
NotifyAdd(addedAmountPooledArray);
GenericObjectPool.Return(addedAmountPooledArray);
}
GenericObjectPool.Return(pooledArray);
//Don't return pooledArray2 because it is now used by m_CurrencyAmounts
return true;
}
Can you tell me what can I do to fix this?
Inventory System version 1.1.7