rhys_vdw
New member
This is the script I use to initialize nodes in my BT.
This is the script that causes a problem:
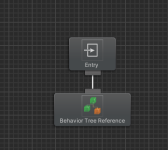
In editor this works as expected, iterating through all the nodes in the referenced behavior:
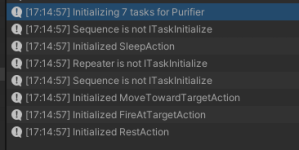
But in build I only get one node (the reference node):
I have yet to work out which part of the code that is causing this.
Code:
public void Initialize(
ExternalBehaviorTree behavior,
int entityId,
GameState gameState,
string name
) {
gameObject.name = $"{entityId}:{name}:{nameof(BehaviorTree)}";
_behaviorTree.ExternalBehavior = behavior;
var tasks = _behaviorTree.FindTasks<Task>();
Debug.Log($"Initializing {tasks.Count} tasks for {name}");
foreach (var task in tasks) {
if (task is ITaskInitialize init) {
init.Initialize(entityId, gameState);
Debug.Log($"Initialized {task.GetType().Name}");
} else {
Debug.Log($"{task.GetType().Name} is not ITaskInitialize");
}
}
}
This is the script that causes a problem:
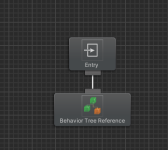
In editor this works as expected, iterating through all the nodes in the referenced behavior:
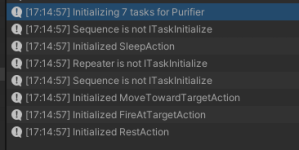
But in build I only get one node (the reference node):
Code:
Initializing 1 tasks for Purifier
UnityEngine.DebugLogHandler:LogFormat(LogType, Object, String, Object[])
UnityEngine.Logger:Log(LogType, Object)
UnityEngine.Debug:Log(Object)
EffortStar.ActorBehavior:Initialize(ExternalBehaviorTree, Int32, GameState, String) (at C:\Users\rhysv\Projects\enter-the-chronosphere\Assets\_Game\Scripts\ActorBehavior.cs:28)
...
BehaviorTreeReference is not ITaskInitialize
UnityEngine.DebugLogHandler:LogFormat(LogType, Object, String, Object[])
UnityEngine.Logger:Log(LogType, Object)
UnityEngine.Debug:Log(Object)
EffortStar.ActorBehavior:Initialize(ExternalBehaviorTree, Int32, GameState, String) (at C:\Users\rhysv\Projects\enter-the-chronosphere\Assets\_Game\Scripts\ActorBehavior.cs:34)
...
I have yet to work out which part of the code that is causing this.