snarlynarwhal
New member
I am having trouble setting up my behavior tree and I think it's because I do not fully understand how hierarchical conditional aborts work.
Intended Behavior
If an enemy is within `Buffer` units from their target, the enemy should back up (Flee to `Buffer` distance) before attacking. In this case, the Observer enemy should back up 3 units before firing it's laser beam, but it does not (you can see the player character's blue hair behind the Observer). It works as intended 90% of the time, but occasionally, it gets "stuck" in the following scenario:
Unintended Behavior
Occasionally the enemy gets "stuck" next to the target and does not back up. Instead it continues to attack without backing up (Fleeing to `Buffer` distance).
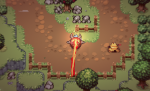
This portion of the behavior tree handles attack. `Buffer` that defines how much space to leave between themselves and the target. The leftmost branch (`Inverter` + `Check Distance`) makes sure that they're not within `Buffer` distance of the target. If they are, they flee to buffer distance in the middle branch (`Sequencer` + `Check Distance` + `Flee`). The rightmost branch makes sure they're still in range before attacking (`Sequencer` + `Check Distance` + `Attack Target`).
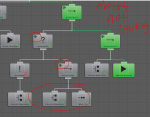
My Understanding
From what I understand from conditional aborts, I would expect the entire left branch to continue to be re-evaluated in the background. I would expect the `Selector` to start with the leftmost `Check Distance`, which would return `Success` since the enemy is within `Buffer` distance from the player. Then the `Inverter` would flip this to a `Failure` causing the `Selector` to go to the `Sequencer` (middle). This second `Check Distance` is the same as the first, so should also return `Success` which should finally lead to the `Flee` task. Again, it functions like this most of the time. Not sure exactly what causes it to get in the "stuck" state.
Intended Behavior
If an enemy is within `Buffer` units from their target, the enemy should back up (Flee to `Buffer` distance) before attacking. In this case, the Observer enemy should back up 3 units before firing it's laser beam, but it does not (you can see the player character's blue hair behind the Observer). It works as intended 90% of the time, but occasionally, it gets "stuck" in the following scenario:
Unintended Behavior
Occasionally the enemy gets "stuck" next to the target and does not back up. Instead it continues to attack without backing up (Fleeing to `Buffer` distance).
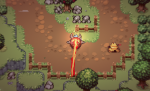
This portion of the behavior tree handles attack. `Buffer` that defines how much space to leave between themselves and the target. The leftmost branch (`Inverter` + `Check Distance`) makes sure that they're not within `Buffer` distance of the target. If they are, they flee to buffer distance in the middle branch (`Sequencer` + `Check Distance` + `Flee`). The rightmost branch makes sure they're still in range before attacking (`Sequencer` + `Check Distance` + `Attack Target`).
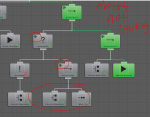
My Understanding
From what I understand from conditional aborts, I would expect the entire left branch to continue to be re-evaluated in the background. I would expect the `Selector` to start with the leftmost `Check Distance`, which would return `Success` since the enemy is within `Buffer` distance from the player. Then the `Inverter` would flip this to a `Failure` causing the `Selector` to go to the `Sequencer` (middle). This second `Check Distance` is the same as the first, so should also return `Success` which should finally lead to the `Flee` task. Again, it functions like this most of the time. Not sure exactly what causes it to get in the "stuck" state.