SamuraiRabbit
New member
Hello there!
I am very new to coding, so the mistakes I have made may be elementary. I'm using the Tactical pack with A*Pathfinding integration for a top-down 2D game. Here is my enemy behaviour tree:
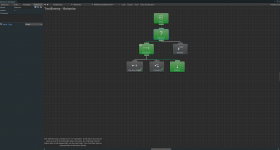
As you can see, it goes through to the attack node, but the attack function is not firing. I used the same code for my player melee attack (which works fine) but my test enemy does not damage the player. Could you help troubleshoot this please?
Here is my Enemy AI script (which implements the IAttackAgent):
And my Player Health script (Which implements the IDamageable):
None of the Debug.Log statements are firing either.
I love what I've achieved with Behaviour Designer so far, and this is the last piece of the puzzle I need for me being happy with my AI.
Thank you in advance.
I am very new to coding, so the mistakes I have made may be elementary. I'm using the Tactical pack with A*Pathfinding integration for a top-down 2D game. Here is my enemy behaviour tree:
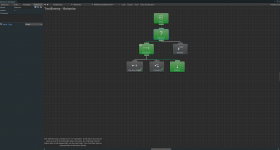
As you can see, it goes through to the attack node, but the attack function is not firing. I used the same code for my player melee attack (which works fine) but my test enemy does not damage the player. Could you help troubleshoot this please?
Here is my Enemy AI script (which implements the IAttackAgent):
C#:
using UnityEngine;
namespace BehaviorDesigner.Runtime.Tactical
{
public class EnemyAI : MonoBehaviour, IAttackAgent
{
public GameObject meleeEffectPrefab;
public Transform attackPoint;
public float attackDistance = 10, repeatAttackDelay = 0.5f, attackAngle = 90, attackDamage = 50;
private float _lastAttackTime;
public LayerMask entityLayers;
private void Awake()
{
_lastAttackTime = -repeatAttackDelay;
}
public float AttackDistance()
{
return attackDistance;
}
public bool CanAttack()
{
return true;
}
public float AttackAngle()
{
return attackAngle;
}
public void Attack(Vector3 targetPosition)
{
Debug.Log("Attacking");
{
GameObject meleeEffect = Instantiate(meleeEffectPrefab, attackPoint.position, attackPoint.rotation);
Destroy(meleeEffect, 0.1f);
Collider2D[] hitTargets = Physics2D.OverlapCircleAll(attackPoint.position, attackDistance, entityLayers);
foreach (Collider2D player in hitTargets)
{
if (player == null)
return;
player.GetComponent<IDamageable>().Damage(attackDamage);
}
}
}
}
}
And my Player Health script (Which implements the IDamageable):
Code:
using UnityEngine;
namespace BehaviorDesigner.Runtime.Tactical
{
public class PlayerHealth : MonoBehaviour, IDamageable
{
[SerializeField] private float _maxhealth = 100f, _currentHealth;
[SerializeField] private UIManager _uIManager;
public GameObject deathEffect;
public void Awake()
{
_currentHealth = _maxhealth;
}
public void Damage(float amount)
{
_currentHealth -= amount;
Debug.Log("Taken Damage!");
_uIManager.UpdateHealth(_currentHealth);
if (_currentHealth <= 0)
{
Die();
}
}
public bool IsAlive()
{
return _currentHealth > 0;
}
void Die()
{
deathEffect = Instantiate(deathEffect, transform.position, Quaternion.identity);
Destroy(deathEffect, 0.1f);
Destroy(gameObject);
}
}
}
None of the Debug.Log statements are firing either.
I love what I've achieved with Behaviour Designer so far, and this is the last piece of the puzzle I need for me being happy with my AI.
Thank you in advance.