macusernick
New member
UPDATE: Please see my last two posts for a working solution!
I've been messing with the AlignToGround and AlignToGravity and noticed that when I make calls to start each respective ability, that they both indicate as starting the AlignToGround ability in the inspector. Also, AlignToGravity is not actually available in the list in the inspector.
I'm trying to start these abilities through code (UCL is a reference to UltimateCharacterLocomotion):
or
I've also noticed that if you fall off an object with the AlignToGround and there is no object below the ground aligned gravity (i.e. when you are upside down), that you will keep falling forever. This makes sense and is the reason I'm trying to get AlignToGravity working correctly. Basically, I'm checking anytime the player Falls to see what is below the Player... if it is nothing (I check 4m) then I attempt to AlignToGravity to flip the player the correct way and fall to the real ground. What's odder is it seems like the AlignToGravity works (even though the AlignToGround says it's active), but it only works when the character is completely up-side down. If the player is only at a 90 degree angle, the system freaks out. Any thoughts? I've put some screenshots below to help illustrate my question/issue.
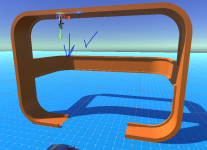
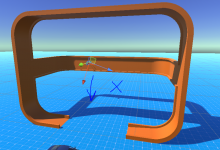
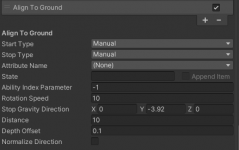
I've been messing with the AlignToGround and AlignToGravity and noticed that when I make calls to start each respective ability, that they both indicate as starting the AlignToGround ability in the inspector. Also, AlignToGravity is not actually available in the list in the inspector.
I'm trying to start these abilities through code (UCL is a reference to UltimateCharacterLocomotion):
Code:
UCL.TryStartAbility(UCL.GetAbility<AlignToGround>());
Code:
UCL.TryStartAbility(UCL.GetAbility<AlignToGravity>());
I've also noticed that if you fall off an object with the AlignToGround and there is no object below the ground aligned gravity (i.e. when you are upside down), that you will keep falling forever. This makes sense and is the reason I'm trying to get AlignToGravity working correctly. Basically, I'm checking anytime the player Falls to see what is below the Player... if it is nothing (I check 4m) then I attempt to AlignToGravity to flip the player the correct way and fall to the real ground. What's odder is it seems like the AlignToGravity works (even though the AlignToGround says it's active), but it only works when the character is completely up-side down. If the player is only at a 90 degree angle, the system freaks out. Any thoughts? I've put some screenshots below to help illustrate my question/issue.
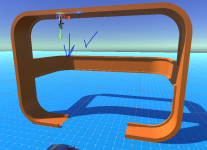
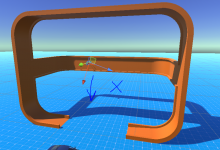
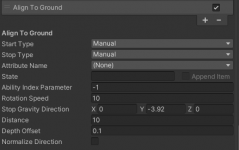
Last edited: