Hi, Andrew
This is going to be a bit of a long one, sorry. I'm working through my custom spear throw and have come to another road block. I would like to be able to Equip and Unequip my Spear (Shootable Item) when I only have one piece of ammo but can not figure out how to do this. I will email a link to a project to you privately to demonstrate this.
In the demo scene, I have a Bow (Shootable Item) item that is first added to the player's inventory. When the player picks up a single Arrow (ammo) the Bow (Shootable Item) is equipped. I am able to fire the weapon at this point. However, if I unequip the Bow (Shootable Item) I am unable to equip it again because on the Bow (Shootable Item) I have "Can Equip On Empty" set to false because I only want the bow to be equippable if they have an arrpw in their inventory. The issue is that while they have picked up an arrow (and technically have one), it is not registered in their inventory because it is loaded into the Bow (Shootable Item).
If, however, I collect a second Arrow, I can equip and unequip my Bow as desired.
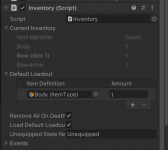
The left image shows the inventory after a single arrow has been collected and the second image shows after a second has been collected
I looked around and see that it can be found with m_LoadedCount of the ItemMonitor script, but from what I can see that can only be read if that specific Item is active - I need to access that Loaded Value of the Bow when the item is not active so I can determine if the Bow can be equipped.
The other issue with this is that I am trying to Unequip the Bow (Shootable Item) if the ammo count is 0. I am using the "OnItemUseConsumableItemIdentifier" event you suggested last week. This is where I am at with that:
I have it kind of working but there are a few issues. First, if I try to Unequip the Bow Item Set (Item Set 6) with the above code it doesn't work . If I instead Equip the Body* (Item Set 20) it works in that it unequips the Bow Item Set but I get the following error related to the "Use" Ability.
I hope this isn't a pain for you to answer. I've been trying to work through it but I stumped.
*I have set the Body Item Set to "Can Switch To" so I could scroll the bow from equipped to unqueipped.
This is going to be a bit of a long one, sorry. I'm working through my custom spear throw and have come to another road block. I would like to be able to Equip and Unequip my Spear (Shootable Item) when I only have one piece of ammo but can not figure out how to do this. I will email a link to a project to you privately to demonstrate this.
In the demo scene, I have a Bow (Shootable Item) item that is first added to the player's inventory. When the player picks up a single Arrow (ammo) the Bow (Shootable Item) is equipped. I am able to fire the weapon at this point. However, if I unequip the Bow (Shootable Item) I am unable to equip it again because on the Bow (Shootable Item) I have "Can Equip On Empty" set to false because I only want the bow to be equippable if they have an arrpw in their inventory. The issue is that while they have picked up an arrow (and technically have one), it is not registered in their inventory because it is loaded into the Bow (Shootable Item).
If, however, I collect a second Arrow, I can equip and unequip my Bow as desired.
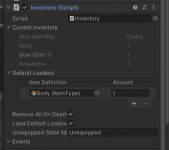
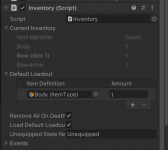
The left image shows the inventory after a single arrow has been collected and the second image shows after a second has been collected
I looked around and see that it can be found with m_LoadedCount of the ItemMonitor script, but from what I can see that can only be read if that specific Item is active - I need to access that Loaded Value of the Bow when the item is not active so I can determine if the Bow can be equipped.
The other issue with this is that I am trying to Unequip the Bow (Shootable Item) if the ammo count is 0. I am using the "OnItemUseConsumableItemIdentifier" event you suggested last week. This is where I am at with that:
Code:
private EquipUnequip[] unequip;
public int itemSetValue = 20;
public void Awake()
{
EventHandler.RegisterEvent<Item, IItemIdentifier, int>(gameObject, "OnItemUseConsumableItemIdentifier", OnItemUseConsumableItemIdentifier);
}
private void Start()
{
unequip = gameObject.GetComponent<UltimateCharacterLocomotion>().GetAbilities<EquipUnequip>();
}
private void OnItemUseConsumableItemIdentifier(Item item, IItemIdentifier identifier, int amount)
{
print(item + " item");
print(identifier + " identifier");
print(amount + " amount");
if (amount == 0 && identifier.ToString() == "BowArrow")
{
for (int i = 0; i < unequip.Length; ++i)
{
if (unequip[i].ItemSetCategoryIndex == 0)
{
print("Try unequip " + unequip[i].ItemSetCategoryIndex.ToString());
unequip[i].StartEquipUnequip(itemSetValue, true, false);
return;
}
}
}
}
public void Destroy()
{
EventHandler.RegisterEvent<Item, IItemIdentifier, int>(gameObject, "OnItemUseConsumableItemIdentifier", OnItemUseConsumableItemIdentifier);
}
I have it kind of working but there are a few issues. First, if I try to Unequip the Bow Item Set (Item Set 6) with the above code it doesn't work . If I instead Equip the Body* (Item Set 20) it works in that it unequips the Bow Item Set but I get the following error related to the "Use" Ability.
Code:
NullReferenceException: Object reference not set to an instance of an object
Opsive.UltimateCharacterController.Character.Abilities.Items.Use.CanStopAbility () (at Assets/Opsive/UltimateCharacterController/Scripts/Character/Abilities/Items/Use.cs:825)
Opsive.UltimateCharacterController.Character.UltimateCharacterLocomotion.TryStopAbility (Opsive.UltimateCharacterController.Character.Abilities.Ability ability, System.Boolean force) (at Assets/Opsive/UltimateCharacterController/Scripts/Character/UltimateCharacterLocomotion.cs:1419)
Opsive.UltimateCharacterController.Character.UltimateCharacterLocomotion.TryStopAbility (Opsive.UltimateCharacterController.Character.Abilities.Ability ability) (at Assets/Opsive/UltimateCharacterController/Scripts/Character/UltimateCharacterLocomotion.cs:1401)
Opsive.UltimateCharacterController.Character.UltimateCharacterLocomotionHandler.TryStopAbility (Opsive.UltimateCharacterController.Character.Abilities.Ability ability) (at Assets/Opsive/UltimateCharacterController/Scripts/Character/UltimateCharacterLocomotionHandler.cs:245)
Opsive.UltimateCharacterController.Character.UltimateCharacterLocomotionHandler.UpdateAbilityInput (Opsive.UltimateCharacterController.Character.Abilities.Ability[] abilities) (at Assets/Opsive/UltimateCharacterController/Scripts/Character/UltimateCharacterLocomotionHandler.cs:122)
Opsive.UltimateCharacterController.Character.UltimateCharacterLocomotionHandler.UpdateAbilityInput () (at Assets/Opsive/UltimateCharacterController/Scripts/Character/UltimateCharacterLocomotionHandler.cs:99)
Opsive.UltimateCharacterController.Character.UltimateCharacterLocomotionHandler.Update () (at Assets/Opsive/UltimateCharacterController/Scripts/Character/UltimateCharacterLocomotionHandler.cs:73)
I hope this isn't a pain for you to answer. I've been trying to work through it but I stumped.
*I have set the Body Item Set to "Can Switch To" so I could scroll the bow from equipped to unqueipped.