SlimDoughnut
New member
In my Reload function, I'm trying to update my attributeValueText value so it updates to its new value after being called. The problem is the Debug log shows that the value has changed but the UI text never updates and stays the same value no matter how times it's called. I feel like it has something to do with SetValue() but I don't know how I should change it to make it work.
Example of value on magazine not changing:
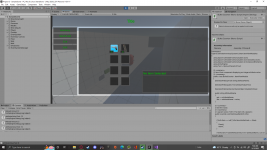
C#:
public class BulletCounter : ItemViewModule
{
[SerializeField] private string attributeName;
[SerializeField] public Text attributeValueText;
[SerializeField] protected GameObject disableOnClear;
public string AttributeName
{
get => attributeName;
set => attributeName = value;
}
/// <summary>
/// Set the value.
/// </summary>
/// <param name="info">The item info.</param>
public override void SetValue(ItemInfo info)
{
if (info.Item == null || info.Item.IsInitialized == false)
{
Clear();
return;
}
if (!info.Item.TryGetAttributeValue<int>(attributeName, out var value))
{
Clear();
return;
}
if (disableOnClear != null)
{
disableOnClear.SetActive(true);
}
attributeValueText.text = $" {info.Amount * value}";
}
public void Reload(ItemInfo info)
{
var pistol = info.Inventory.gameObject.GetComponentInChildren<Pistol>();
var inventoryGrid = InventorySystemManager.GetItemViewSlotContainer<InventoryGrid>(1, "My Inventory Grid Panel");
int ammo = int.Parse(attributeValueText.text);
attributeValueText.text = $" {ammo - pistol.reloadAmount}";
inventoryGrid.Draw();
Debug.Log(pistol.reloadAmount);
Debug.Log(attributeValueText.text);
}
/// <summary>
/// Clear the value.
/// </summary>
public override void Clear()
{
if (disableOnClear != null)
{
disableOnClear.SetActive(false);
}
}
}
}
Example of value on magazine not changing:
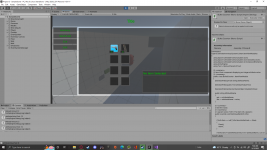