I have an event which I want to send to the tree on start:
If I put this code into Start, the tree does not receive the event (I checked with a Log task). I have to put it into Update instead before the event works.
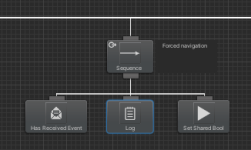
Code:
private void Start()
{
if (InitialDestination.HasValue)
{
Debug.Log($"sending destination {InitialDestination.Value}");
_behaviorTree.SendEvent<object>("RecievedDestination", InitialDestination.Value);
InitialDestination = null;
}
}
If I put this code into Start, the tree does not receive the event (I checked with a Log task). I have to put it into Update instead before the event works.
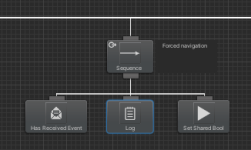
Last edited: