dangoyette
New member
I'm struggling with how to structure my Behavior Tree to allow for complex conditions without interrupting long-running actions. What I'm finding is that any Success in a Conditional node will trigger a conditional abort on the currently running task, even if the rest of the branch doesn't result in Success.
Here's a simple example:
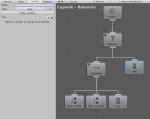
On the left, we have a pair of Compare Shared Bool nodes, with inverted comparisons. It's impossible the them both to return Success. One will always return Failure, regardless of the value of Val1. When I start running, one of those two will fail, and we'll happily enter the "Idle" node on the right, which just returns "Running" indefinitely, and the tree is stable.
However, if I toggle the value of Val1, the "Idle" node will be aborted, as the results of "Val1 is TRUE" and "Val1 is FALSE" will switch output. However, the value of their parent Sequence remains Failure, since one of the two will definitely fail. But the damage has been done. "Idle" has been interrupted, and will restart. If "Idle" were some other long-running process, this becomes a problem.
I thought maybe my approach to evaluating conditions was just not the right way of going about things. I instead tried replacing the Compare Shared Bool with a pair of "Conditional Evaluators", which themselves perform the Shared Bool comparison, one against True and one against False. Although this runs on the first tick, it doesn't reevaluate when "Val1" changes, even if I choose "Reevaluate" on the node. Someone reported this issue in this thread: (https://www.opsive.com/forum/index....-again-while-another-subtree-is-running.1505/) However, there wasn't a clear answer. Instead, the user was told to use plain old conditional nodes.
Is there another way to structure my logic above such that "Val1" can change without aborting "Idle"? I realize I could put all of my logic into a single custom node, but that means I can no longer compose my tree from small, reusable nodes.
So, to recap, I was hoping to be able to chain together conditional nodes to control aborts. For example, "Perform X if A, B, and C are all true, otherwise perform Y". The way it works now, if "Y" is running, and any of conditions A, B, or C return Success, "Y" will be aborted, even if A, B, and C aren't all true. This results in Y immediately restarting, rather than remaining in a continuous running state.
Here's a simple example:
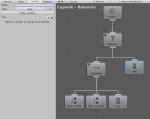
On the left, we have a pair of Compare Shared Bool nodes, with inverted comparisons. It's impossible the them both to return Success. One will always return Failure, regardless of the value of Val1. When I start running, one of those two will fail, and we'll happily enter the "Idle" node on the right, which just returns "Running" indefinitely, and the tree is stable.
However, if I toggle the value of Val1, the "Idle" node will be aborted, as the results of "Val1 is TRUE" and "Val1 is FALSE" will switch output. However, the value of their parent Sequence remains Failure, since one of the two will definitely fail. But the damage has been done. "Idle" has been interrupted, and will restart. If "Idle" were some other long-running process, this becomes a problem.
I thought maybe my approach to evaluating conditions was just not the right way of going about things. I instead tried replacing the Compare Shared Bool with a pair of "Conditional Evaluators", which themselves perform the Shared Bool comparison, one against True and one against False. Although this runs on the first tick, it doesn't reevaluate when "Val1" changes, even if I choose "Reevaluate" on the node. Someone reported this issue in this thread: (https://www.opsive.com/forum/index....-again-while-another-subtree-is-running.1505/) However, there wasn't a clear answer. Instead, the user was told to use plain old conditional nodes.
Is there another way to structure my logic above such that "Val1" can change without aborting "Idle"? I realize I could put all of my logic into a single custom node, but that means I can no longer compose my tree from small, reusable nodes.
So, to recap, I was hoping to be able to chain together conditional nodes to control aborts. For example, "Perform X if A, B, and C are all true, otherwise perform Y". The way it works now, if "Y" is running, and any of conditions A, B, or C return Success, "Y" will be aborted, even if A, B, and C aren't all true. This results in Y immediately restarting, rather than remaining in a continuous running state.