imaethan
New member
So I've been testing performance recently and noticed in the profiler that when there's a decent number of enemies enabled the performance tanks, due to BehaviorManager.Update(), it's 30 - 40% of Update.ScriptRunBehaviourUpdate.
Any tips on optimising my behaviour trees? I've attached a screenshot below.
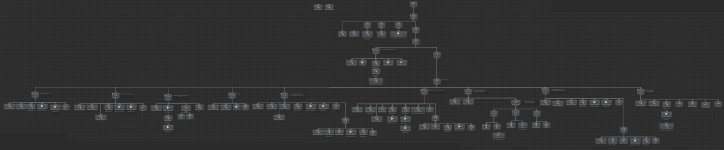
I've also attached a screenshot of the profiler, before and after I disable the enemies.
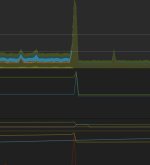
Any help or suggestions would be much appreciated.
Any tips on optimising my behaviour trees? I've attached a screenshot below.
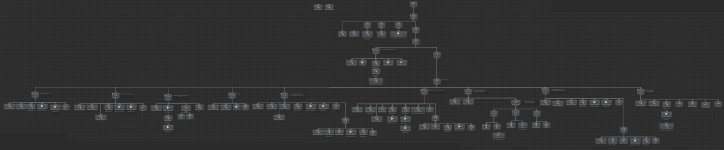
I've also attached a screenshot of the profiler, before and after I disable the enemies.
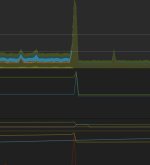
Any help or suggestions would be much appreciated.