Evgenij Tsvetkov
Member
Hi!
You can use the Modify expression syntax to get to the attributes of the nested ItemDefinition. We want to try to make weapons modular, I make Attribute<ItemDefenition> and replace it at runtime. Do you think it will be possible to get to the attributes of this item in order to write an auto calculation of the parameters of the main item?
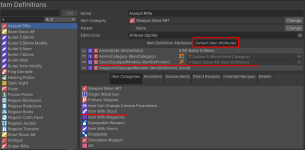
As an example, there is the weight of the main item, the module has been invested, it also has a weight. Automatically calculated weights based on Modify Syntax
[Weight] + StockEquippedModule[Weight] + MagazineEquippedModule[Weight]
You can use the Modify expression syntax to get to the attributes of the nested ItemDefinition. We want to try to make weapons modular, I make Attribute<ItemDefenition> and replace it at runtime. Do you think it will be possible to get to the attributes of this item in order to write an auto calculation of the parameters of the main item?
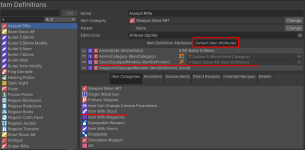
As an example, there is the weight of the main item, the module has been invested, it also has a weight. Automatically calculated weights based on Modify Syntax
[Weight] + StockEquippedModule[Weight] + MagazineEquippedModule[Weight]
Last edited: