skinwalker
Member
Hi,
I already got the currency working, but with inventories there are a few issues.
These are all my item collections
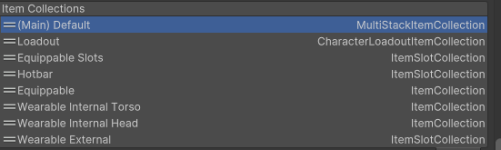
CODE :
1) I'd like to save them at different times not all at once so the question is about this script InventorySystemManagerItemSaver. Should I explicitely save it because I see it has a key too or it saves and loads automatically as long as it is in the scene and I use the code above to save a saver by key? I don't see any results when I load the items thats why I ask what should I do with that script. If I must save that saver too the question is when? I save my other savers at different time, should I save it with every saver in every saver's save file, then load it with every saver?
2) InventoryBridgeSaver - Does that save the Equippable Slots only? (Loadout too if it makes sense) or even if you have other collections not related to UCC it will save them so InventorySaver is not needed?
3) Looking at the image above I think I need for the UI part of saving:
(Main) Default - ItemShapeGridDataSaver
-EquippableSlots - ??
-Hotbar - ??
-Equippable - ??
-Wearable Internal Torso - ItemShapeGridDataSaver
-Wearable Internal Head - ItemShapeGridDataSaver
-Wearable External - ??
Stash / Storage inventory uses ItemShapeGridDataSaver + InventorySaver
I save and load normally during 1 play session in the menu scene, when I quit the game and restart the items never load its just empty collection.
3) Is it possible loading broken saves to break your item definitions inside the database? A few times I see my stackable item defs losing their parent and I gotta manually assign it in the database after loading a broken save while experimenting.
I have so many questions and no answers in the documentations nor the demos.
I already got the currency working, but with inventories there are a few issues.
These are all my item collections
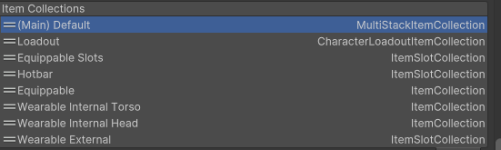
CODE :
private byte[] GetSaverSerializedData(string saverKey)
{
SaveSystemManager.Save(saveSlot, false);
var isDataRetrieved = SaveSystemManager.TryGetSaveData(saverKey, out Serialization serializedData);
var data = ES3.Serialize(serializedData);
return data;
}
private void LoadSaver(byte[] data)
{
var saveData = ES3.Deserialize<Serialization>(data);
var s = FindObjectsOfType<SaverBase>().FirstOrDefault(s => s.FullKey.Equals(StashSaverKey));
s.DeserializeAndLoadSaveData(saveData);
}
[Button]
public void Save()
{
var dataToSave = SaveSaver(StashSaverKey);
ES3.Save(InventoriesSaveFile.StashKey, dataToSave, GetStashLocation());
}
[Button]
public void Load()
{
var data = ES3.Load<byte[]>(InventoriesSaveFile.StashKey, GetStashLocation());
LoadSaver(data);
}
1) I'd like to save them at different times not all at once so the question is about this script InventorySystemManagerItemSaver. Should I explicitely save it because I see it has a key too or it saves and loads automatically as long as it is in the scene and I use the code above to save a saver by key? I don't see any results when I load the items thats why I ask what should I do with that script. If I must save that saver too the question is when? I save my other savers at different time, should I save it with every saver in every saver's save file, then load it with every saver?
2) InventoryBridgeSaver - Does that save the Equippable Slots only? (Loadout too if it makes sense) or even if you have other collections not related to UCC it will save them so InventorySaver is not needed?
3) Looking at the image above I think I need for the UI part of saving:
(Main) Default - ItemShapeGridDataSaver
-EquippableSlots - ??
-Hotbar - ??
-Equippable - ??
-Wearable Internal Torso - ItemShapeGridDataSaver
-Wearable Internal Head - ItemShapeGridDataSaver
-Wearable External - ??
Stash / Storage inventory uses ItemShapeGridDataSaver + InventorySaver
I save and load normally during 1 play session in the menu scene, when I quit the game and restart the items never load its just empty collection.
3) Is it possible loading broken saves to break your item definitions inside the database? A few times I see my stackable item defs losing their parent and I gotta manually assign it in the database after loading a broken save while experimenting.
I have so many questions and no answers in the documentations nor the demos.
Last edited: