I am trying to create a cooldown task. When the task is first visited in the behavior tree it should execute its child as normally, then if the child's TaskStatus was a success and/or failure, a cooldown timer should begin and the cooldown task should not allow its child to execute again, returning TaskStatus.Failure, until the timer reaches zero. Here is what I have right now:
public class CooldownTimer : Decorator
{
public float cooldownTime;
public bool cooldownStartOnSuccess;
public bool cooldownStartOnFailure;
private float cooldownStart;
public override bool CanExecute() {
return (Time.time - cooldownStart >= cooldownTime) ? true : false;
}
public override void OnChildExecuted(TaskStatus childStatus) {
if (cooldownStartOnSuccess && childStatus == TaskStatus.Success) cooldownStart = Time.time;
if (cooldownStartOnFailure && childStatus == TaskStatus.Failure) cooldownStart = Time.time;
}
}
And here is what my behavior tree looks like right now:
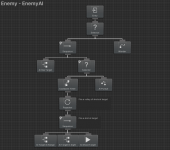
I am trying to get it so that when the enemy spots a player it will chase it until the player is within shooting range and unobstructed by any obstacles then fire a number of shots, and try to close the distance after until it is ready to fire another volley when the cooldown ends. However I'm getting some unexpected problems:
After the enemy fires a volley at the player and the cooldown timer starts, the enemy does not enter the Ai Pursue state and close the distance to the target while the timer counts down.
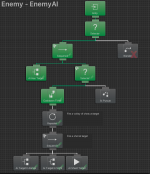
Then there are cases where the enemy is shooting at the player but the player moves out of range or sight, and the enemy pursues the player but never tries shooting again even when the player comes back into sight and range. Here's the state of the behavior tree at one of those times.
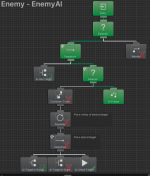
I'm burnt out on trying to figure out where I went wrong right now, and I'm having trouble finding any further documentation on the base class Decorator that might help me understand where my errors lie. I'm sure there's something simple I'm forgetting; I only just started using Behavior Designer about a week ago and there's a lot I still don't fully understand. If someone sees what I'm missing from my custom task please let me know, or better yet, if you have your own solution to building a cooldown timer please do share.
public class CooldownTimer : Decorator
{
public float cooldownTime;
public bool cooldownStartOnSuccess;
public bool cooldownStartOnFailure;
private float cooldownStart;
public override bool CanExecute() {
return (Time.time - cooldownStart >= cooldownTime) ? true : false;
}
public override void OnChildExecuted(TaskStatus childStatus) {
if (cooldownStartOnSuccess && childStatus == TaskStatus.Success) cooldownStart = Time.time;
if (cooldownStartOnFailure && childStatus == TaskStatus.Failure) cooldownStart = Time.time;
}
}
And here is what my behavior tree looks like right now:
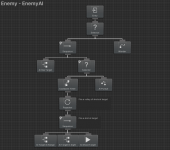
I am trying to get it so that when the enemy spots a player it will chase it until the player is within shooting range and unobstructed by any obstacles then fire a number of shots, and try to close the distance after until it is ready to fire another volley when the cooldown ends. However I'm getting some unexpected problems:
After the enemy fires a volley at the player and the cooldown timer starts, the enemy does not enter the Ai Pursue state and close the distance to the target while the timer counts down.
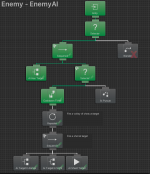
Then there are cases where the enemy is shooting at the player but the player moves out of range or sight, and the enemy pursues the player but never tries shooting again even when the player comes back into sight and range. Here's the state of the behavior tree at one of those times.
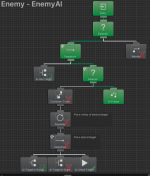
I'm burnt out on trying to figure out where I went wrong right now, and I'm having trouble finding any further documentation on the base class Decorator that might help me understand where my errors lie. I'm sure there's something simple I'm forgetting; I only just started using Behavior Designer about a week ago and there's a lot I still don't fully understand. If someone sees what I'm missing from my custom task please let me know, or better yet, if you have your own solution to building a cooldown timer please do share.