Hi,
I am trying to setup a damage receiver on an emerald ai mob that I picked up somewhere
I've placed the code on the mob in the parent object
I have a breakpoint right before it does the call and all parameters seem to be good, none of them have null values
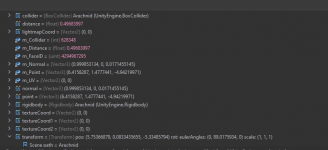
It boggles my mind why I would get a NullReferenceException
Any idea what might be wrong? I have not set the mob up as an ai agent, when I did it kept falling through the floor, does the mob need to have the opsive agent components for this to work? If so how do I prevent the mob from falling down?
I am trying to setup a damage receiver on an emerald ai mob that I picked up somewhere
Code:
using Opsive.Shared.Events;
using UnityEngine;
using Opsive.UltimateCharacterController.Events;
using EmeraldAI;
public class OpsiveBridge : MonoBehaviour
{
private EmeraldAISystem _emeraldAI;
private void Awake()
{
_emeraldAI = GetComponent<EmeraldAISystem>();
Opsive.Shared.Events.EventHandler.RegisterEvent<float, Vector3, Vector3, GameObject, Collider>(gameObject,
"OnObjectImpact", OnObjectImpact);
}
private void OnObjectImpact(float amount, Vector3 point, Vector3 normal, GameObject
originator, Collider collider)
{
if (_emeraldAI != null)
{
_emeraldAI.Damage((int)amount, EmeraldAISystem.TargetType.Player);
//Debug.Log(this.name + " took " + amount + " damaged at location " + point + "with normal " + normal + " from object " + originator);
}
}
}
I've placed the code on the mob in the parent object
I have a breakpoint right before it does the call and all parameters seem to be good, none of them have null values
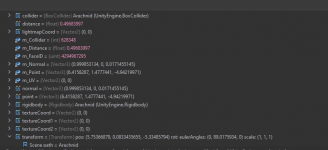
It boggles my mind why I would get a NullReferenceException
Code:
[Exception] NullReferenceException: Object reference not set to an instance of an object
EventHandler.ExecuteEvent[T1,T2,T3,T4,T5,T6]() at <27da9e1afec54f2fb2a11d46a234f9df>:0
ShootableWeapon.HitscanFire() at /Opsive/UltimateCharacterController/Scripts/Items/Actions/ShootableWeapon.cs:982
SchedulerBase.AddEventInternal[T]() at <3261d1d1480d44a1abe2cb945dc5a01c>:0
SchedulerBase.Schedule[T]() at <3261d1d1480d44a1abe2cb945dc5a01c>:0
ShootableWeapon.Fire() at /Opsive/UltimateCharacterController/Scripts/Items/Actions/ShootableWeapon.cs:780
ShootableWeapon.UseItem() at /Opsive/UltimateCharacterController/Scripts/Items/Actions/ShootableWeapon.cs:688
Use.LateUpdate() at /Opsive/UltimateCharacterController/Scripts/Character/Abilities/Items/Use.cs:649
UltimateCharacterLocomotion.LateUpdateActiveAbilities() at /Opsive/UltimateCharacterController/Scripts/Character/UltimateCharacterLocomotion.cs:1042
UltimateCharacterLocomotion.LateUpdateUltimateLocomotion() at /Opsive/UltimateCharacterController/Scripts/Character/UltimateCharacterLocomotion.cs:1028
UltimateCharacterLocomotion.UpdatePositionAndRotation() at /Opsive/UltimateCharacterController/Scripts/Character/UltimateCharacterLocomotion.cs:843
CharacterLocomotion.UpdatePositionAndRotation() at /Opsive/UltimateCharacterController/Scripts/Character/CharacterLocomotion.cs:521
CharacterLocomotion.OnAnimatorMove() at /Opsive/UltimateCharacterController/Scripts/Character/CharacterLocomotion.cs:1459
UltimateCharacterLocomotion.OnAnimatorMove() at /Opsive/UltimateCharacterController/Scripts/Character/UltimateCharacterLocomotion.cs:1771
Any idea what might be wrong? I have not set the mob up as an ai agent, when I did it kept falling through the floor, does the mob need to have the opsive agent components for this to work? If so how do I prevent the mob from falling down?
Last edited: