Haytam95
Active member
I'm implementing my custom health system, and i'm listening for the "OnObjectImpact" event to detect whenever the character gets hit.
Here is my custom script that handles the event:
But the method isn't called.
Here is my debugging:
MeleeWeapon invokes correctly the event
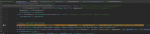
But my custom file (Character Health) isn't getting called
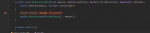
I'm missing something? (I'm not using at all the default Health system)
Here is my custom script that handles the event:
C#:
protected void Awake()
{
propertiesManager = GetComponent<PropertiesManager>();
health = (IProperty<float>) propertiesManager.FindPropertyByName(healthPropertyName);
EventHandler.RegisterEvent<float, Vector3, Vector3, GameObject, object, Collider>(gameObject, "OnObjectImpact",
OnCharacterHit);
}
private void Update()
{
currentHealth = health.GetValue();
}
private void OnCharacterHit(float amount, Vector3 position, Vector3 forceDirection, GameObject attacker,
object attackerObject, Collider hitCollider)
{
print("Damage received");
health.SetValue(health.GetValue() - amount);
}
But the method isn't called.
Here is my debugging:
MeleeWeapon invokes correctly the event
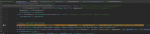
But my custom file (Character Health) isn't getting called
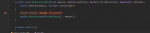
I'm missing something? (I'm not using at all the default Health system)