cptscrimshaw
Member
In version 1.0 I had set up a custom craftinginteractable that referenced a list of crafting recipes that the player had learned and used that to populate the recipe list of the crafting menu. I tried this with version 1.1 by creating a custom Crafter.cs script, but for whatever reason when I try to drag it into Crafting Menu Opener in the inspector, it won't take it. This leads me to believe that there is now a different way of doing this. I looked at the documentation (https://opsive.com/support/documentation/ultimate-inventory-system/crafting/) and it mentions at the bottom that you can edit the list of recipes the crafter accepts (List<CraftingRecipes> recipes = m_Crafter.CraftinRecipes
, but I wasn't able to wrap my head around where I should actually be updating it.
Previously, I did the following in the CraftingInteractableBehavior script:
This leaves me with two questions:
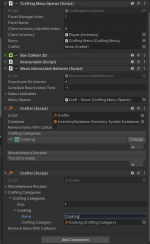

Previously, I did the following in the CraftingInteractableBehavior script:
Code:
protected void SetCraftingRecipes()
{
var recipeList = new List<CraftingRecipe>(m_MiscellanousRecipes);
var characterRecipeList = player.GetComponent<CharacterCraftingData>().myRecipes;
for (int i = 0; i < m_RecipeCategories.Length; i++)
{
var pooledArray = GenericObjectPool.Get<CraftingRecipe[]>();
var recipesCount = m_RecipeCategories[i].GetAllChildrenElements(ref pooledArray);
var characterRecipesCount = characterRecipeList.Count;
for (int j = 0; j < recipesCount; j++)
{
for (int k = 0; k < characterRecipesCount; k++)
{
if (pooledArray[j] == characterRecipeList[k])
{
recipeList.Add(pooledArray[j]);
}
}
}
GenericObjectPool.Return(pooledArray);
}
m_Recipes = recipeList.ToArray();
}
This leaves me with two questions:
- Unfortunately, any edits I do to a custom copy of the Crafter script result in the Crafting Menu Opener not accepting the new Crafter component in the inspector. Any suggestions on what could be causing this? Strangely, my updated Crafter component script looks quite different in the inspector (even if I just change 1 line). Does this have to do with the script existing within my Assets/Scripts folder and the rest of the UIS scripts existing in the Assets/Opsive/... folder? Included is an image with both versions of the Crafter script added as components.
- Is there an easier, better way to have a crafter only accept a specific list of recipes that I give it in v1.1?
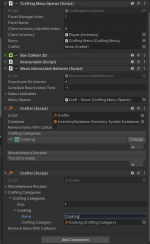