Apologies for the vagueness. I have just a draft of a custom movement type (essentially like point and click with the point and click removed!). I am using my own pathfinding grid and pathfinding solution and navigating that grid with an ability that follows the waypoints. I am driving the movement by calculating an inputvector and rotating the character in UpdateRotation and tweaking the movement in ApplyPosition. I see my issue without any rotation code. Here is ApplyPosition:
In short, when m_ArrivedAtDestination is true (the last waypoint is reached), the code tampers with the MoveDirection to perfectly align the character to the tile and then it stops the ability. However, you will note, I am stopping the ability with the scheduler. This is fine with my use case, but I am not sure why this is necessary? If I stop the ability directly as soon as the criteria are met some extra movement comes from somewhere and the character always overshoots. This still happens with a very short schedule time, but doesnt when the schedule time is increased, eg. maybe above 0.3-0.4 seconds.
Hopefully this clarifies what should happen, correct movement: https://drive.google.com/file/d/1zb5_93eNRvNDh_2wR3isuWCWTICulh5b/view?usp=sharing
you'll see that the movement ends with the character correctly in the tile's centre (character moving down the picture with the grid marking the tiles):
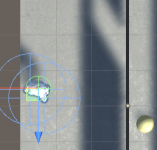
Without a delay it overshoots:
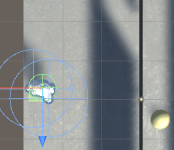
The odd thing is that the debug says the character is in the correct position and hence the MoveDirection is calculated correctly as Vector3.zero, but as soon as the ability stops it drifts that bit forward and the position reports incorrect. (Inputvector is being set as Vector2.zero at this point - when m_ArrivedAtDestination = true).
So the question: Given that I am overriding position and inputvector, what time sensitive thing am i overlooking that is moving the character forward as the ability is stopped? presumably something is running down that i need to zero out manually?
(I appreciate the code can be optimised, but i wanted to understand the working before moving to that)
Thanks
Code:
public override void ApplyPosition() {
Vector3 moveDirection = m_CharacterLocomotion.MoveDirection;
if (m_ArrivedAtDestination) {
moveDirection = m_PathList[m_TargetIndex].transform.position - m_Transform.position;
moveDirection.y = 0;
if (moveDirection.magnitude < 0.01f) {
Scheduler.ScheduleFixed(1f, Stop);
}
}
else if (m_TargetIndex == 0) {
if (moveDirection.magnitude > m_DesiredDirection.magnitude) {
moveDirection = m_DesiredDirection;
}
}
m_CharacterLocomotion.MoveDirection = moveDirection;
}
In short, when m_ArrivedAtDestination is true (the last waypoint is reached), the code tampers with the MoveDirection to perfectly align the character to the tile and then it stops the ability. However, you will note, I am stopping the ability with the scheduler. This is fine with my use case, but I am not sure why this is necessary? If I stop the ability directly as soon as the criteria are met some extra movement comes from somewhere and the character always overshoots. This still happens with a very short schedule time, but doesnt when the schedule time is increased, eg. maybe above 0.3-0.4 seconds.
Hopefully this clarifies what should happen, correct movement: https://drive.google.com/file/d/1zb5_93eNRvNDh_2wR3isuWCWTICulh5b/view?usp=sharing
you'll see that the movement ends with the character correctly in the tile's centre (character moving down the picture with the grid marking the tiles):
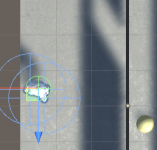
Without a delay it overshoots:
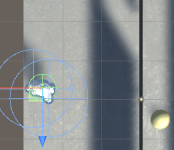
The odd thing is that the debug says the character is in the correct position and hence the MoveDirection is calculated correctly as Vector3.zero, but as soon as the ability stops it drifts that bit forward and the position reports incorrect. (Inputvector is being set as Vector2.zero at this point - when m_ArrivedAtDestination = true).
So the question: Given that I am overriding position and inputvector, what time sensitive thing am i overlooking that is moving the character forward as the ability is stopped? presumably something is running down that i need to zero out manually?
(I appreciate the code can be optimised, but i wanted to understand the working before moving to that)
Thanks