Hi,
I've been testing out my game in the editor using my mouse and keyboard for about two-three weeks now. And I recently thought that I should also make sure things work with a controller.
And turns out my Camera does not move when I use my right joystick, it works perfectly fine when I use the Mouse though. from what I can tell all the other inputs work just fine.
So here is how I got my things set up:
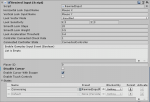
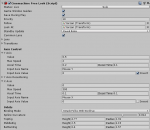
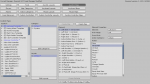
I don't have anything set up to differentiate using a controller or the mouse and keyboard, at least I haven't added anything to the default states.
Could it be that the Mouse X and Y input get reset by the mouse before the Cinemachine Camera gets to move?
Maybe I haven't it up correctly.
Also on a side note, probably isn't related but, when I load up my scene for the first time my character is always running. When I change scenes, or when I load the scene from the title screen, I need to press shift to run. I have no idea why. From what I can tell my character controller is setup to run only when shift is hold down. Not too much of a problem since I plan to have the character always run, I'm just curious.
Thank you for your time,
Santiago
I've been testing out my game in the editor using my mouse and keyboard for about two-three weeks now. And I recently thought that I should also make sure things work with a controller.
And turns out my Camera does not move when I use my right joystick, it works perfectly fine when I use the Mouse though. from what I can tell all the other inputs work just fine.
So here is how I got my things set up:
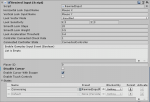
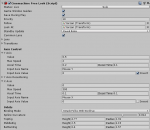
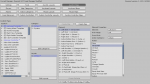
I don't have anything set up to differentiate using a controller or the mouse and keyboard, at least I haven't added anything to the default states.
Could it be that the Mouse X and Y input get reset by the mouse before the Cinemachine Camera gets to move?
Maybe I haven't it up correctly.
Also on a side note, probably isn't related but, when I load up my scene for the first time my character is always running. When I change scenes, or when I load the scene from the title screen, I need to press shift to run. I have no idea why. From what I can tell my character controller is setup to run only when shift is hold down. Not too much of a problem since I plan to have the character always run, I'm just curious.
Thank you for your time,
Santiago