1. Create a new project with Unity 2021.3.13f1.
2. Download and install Third Person Controller 3.0.6.
3. Set the IK Target of the weapon.
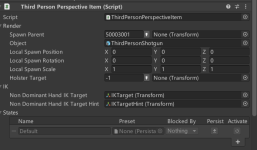
4. For the convenience of testing, modify the code.
UltimateCharacterController\Scripts\Objects\CharacterAssist\ItemPickup.cs
5. Equip the weapon with IK target.
6. Equip another weapon that doesn't exist in your inventory.
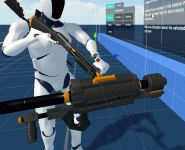
The soft equipped CharacterItem overrides the IK Target of the equipped CharacterItem.
Please fix it and where relevant, thanks!
2. Download and install Third Person Controller 3.0.6.
3. Set the IK Target of the weapon.
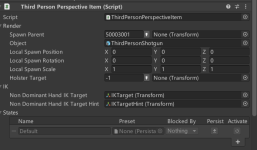
4. For the convenience of testing, modify the code.
UltimateCharacterController\Scripts\Objects\CharacterAssist\ItemPickup.cs
Code:
var itemSetManager = inventory.gameObject.GetCachedComponent<ItemSetManagerBase>();
itemSetManager.UpdateItemSets();
//if (string.IsNullOrWhiteSpace(m_ItemSetName) == false) {
// if (itemSetManager.TryEquipItemSet(m_ItemSetName, m_ItemSetGroup, forceEquip, false)) {
// return true;
// } else {
// Debug.LogWarning($"Cannot equip item set '{m_ItemSetName}' it might be invalid, disabled or might not exist.");
// }
//}
for (int i = 0; i < m_ItemDefinitionAmounts.Length; i++) {
var itemIdentifierAmount = m_ItemDefinitionAmounts[i];
for (int j = inventory.SlotCount - 1; j >= 0; j--) {
var characterItem = inventory.GetCharacterItem(itemIdentifierAmount.ItemIdentifier, j);
if (characterItem == null) { continue; }
// Only equip if the item is not already equipped or about to be equipped.
// It can sometimes be equipping from the Pickup event.
if (itemSetManager.IsItemContainedInActiveItemSet(m_ItemSetGroup, characterItem.ItemIdentifier) ||
itemSetManager.IsItemContainedInNextItemSet(m_ItemSetGroup, characterItem.ItemIdentifier)) {
continue;
}
var itemSet = itemSetManager.GetItemSet(characterItem.ItemIdentifier, m_ItemSetGroup, true);
if (itemSet == null) { continue; }
//itemSetManager.TryEquipItemSet(itemSet, forceEquip, false);
}
}
5. Equip the weapon with IK target.
6. Equip another weapon that doesn't exist in your inventory.
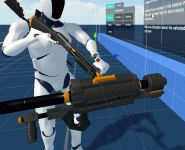
The soft equipped CharacterItem overrides the IK Target of the equipped CharacterItem.
Please fix it and where relevant, thanks!