Haytam95
Active member
Hi !
I'm creating a custom inspector that allows to instance external BD at runtime, in this case it's working with Playmaker but could be in other script as well.
I've created the inspector of PM that allows me to select an External Behavior Tree, when I select one, then the Variables shows up like this:
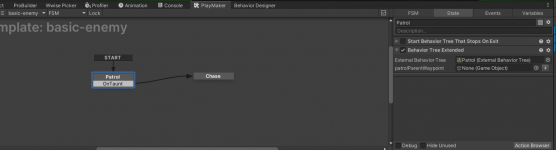
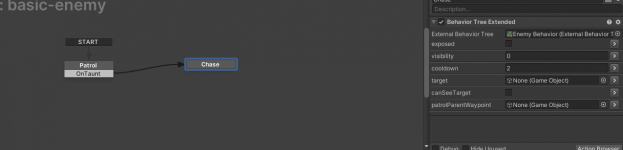
I'm novice at developing inspectors, so I'm stuck in the part of serializing the data (I think). The trouble is that when the game start, the Variables that I've assigned doesn't show up in the game and the inspector gets cleaned.
Here is my Inspector:
And here is my PM task:
How can I fix this?
I'm creating a custom inspector that allows to instance external BD at runtime, in this case it's working with Playmaker but could be in other script as well.
I've created the inspector of PM that allows me to select an External Behavior Tree, when I select one, then the Variables shows up like this:
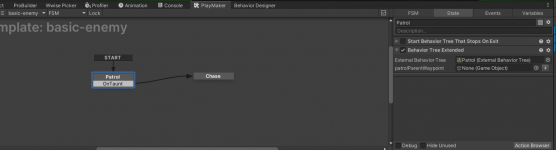
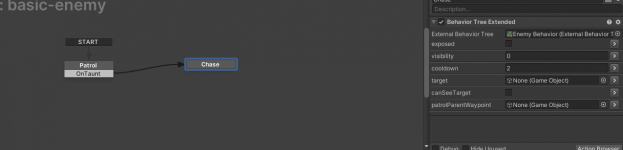
I'm novice at developing inspectors, so I'm stuck in the part of serializing the data (I think). The trouble is that when the game start, the Variables that I've assigned doesn't show up in the game and the inspector gets cleaned.
Here is my Inspector:
C#:
public override bool OnGUI()
{
DrawDefaultInspector();
if (!(target is BehaviorTreeExtended action) || action.ExternalBehaviorTree == null) return true;
// Dibujar datos del Behavior Tree
if (action.SharedVariables == null || action.ExternalBehaviorTree != _externalBehavior)
{
_externalBehavior = action.ExternalBehaviorTree;
action.SharedVariables = action.ExternalBehaviorTree.GetBehaviorSource().GetAllVariables();
}
List<SharedVariable> allVariables = action.ExternalBehaviorTree.GetBehaviorSource().GetAllVariables();;
if (allVariables != null && allVariables.Count > 0)
{
if (VariableInspector.DrawAllVariables(false, action.ExternalBehaviorTree.GetBehaviorSource(), ref allVariables,
false,
ref variablePosition, ref selectedVariableIndex, ref selectedVariableName,
ref selectedVariableTypeIndex,
false,
true))
{
// Si no se está jugando
if (!EditorApplication.isPlayingOrWillChangePlaymode)
{
action.SharedVariables = allVariables;
}
}
}
else
{
EditorGUILayout.LabelField("There are no variables to display");
}
return true;
}
And here is my PM task:
C#:
public class BehaviorTreeExtended : FsmStateAction
{
public ExternalBehaviorTree ExternalBehaviorTree;
private BehaviorSource _behaviorSource = new BehaviorSource();
public BehaviorSource behaviorSource
{
get => _behaviorSource;
set => _behaviorSource = value;
}
public override void OnEnter()
{
base.OnEnter();
var behaviorTree = Owner.AddComponent<BehaviorTree>();
behaviorTree.ExternalBehavior = ExternalBehaviorTree;
behaviorTree.SetBehaviorSource(_behaviorSource);
/*foreach (var variable in _behaviorSource.GetAllVariables())
{
behaviorTree.SetVariable(variable.Name, variable);
}*/
behaviorTree.EnableBehavior();
}
}
How can I fix this?
Last edited: