[EDIT] I posted this in the wrong forum by mistake at first. So I reposted it here. Sorry.[/EDIT]
Hello everyone,
I'm new to behavioir designer and I'm trying to create my first own task. I'm currently trying to alter the patrol task so the agent walks back and forth between the waypoints and not in a circle. So far so good... but I encountered a problem and I just can't wrap my head around what is going wrong...
I copied the code from the patrol task script and renamed the class.
And now I'm trying to feed my waypoints into the task. I have a WayPointManager Script which gives me the list of waypoints in my scene.
At first I tried to call the function on the WayPointManager Script to get the list of waypoints in the OnStart method on the task. As soon as
I start the scene, I get an error message:

Then I added the waypoints variable to the shared variables inside the behaviour designer.
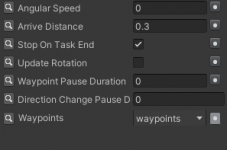
And I added a script to my gameobject which sets the waypoints on Start.
On runtime I can see the waypoints are correctly added to the shared variable. But I still get the same error message from the beginning.
The error messages occures as soon a the task tries to access the waypoints...
When I add the waypoints manually from the scene to the behaviour tree everything works fine... but I need to add the waypoints on runtime, because the gameobject with the bt is a prefab
UPDATE:
I just tried to use the original patrol task from the movement pack and changed assigned the waypoints variable to the shared variable. And then added the waypoints on runtime. I get the same error.
Hello everyone,
I'm new to behavioir designer and I'm trying to create my first own task. I'm currently trying to alter the patrol task so the agent walks back and forth between the waypoints and not in a circle. So far so good... but I encountered a problem and I just can't wrap my head around what is going wrong...
I copied the code from the patrol task script and renamed the class.
And now I'm trying to feed my waypoints into the task. I have a WayPointManager Script which gives me the list of waypoints in my scene.
At first I tried to call the function on the WayPointManager Script to get the list of waypoints in the OnStart method on the task. As soon as
I start the scene, I get an error message:

Then I added the waypoints variable to the shared variables inside the behaviour designer.
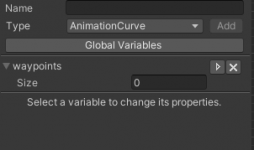
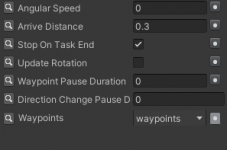
And I added a script to my gameobject which sets the waypoints on Start.
C#:
public class EnemyController : MonoBehaviour{
void Start()
{
BehaviorTree behaviourTree = GetComponent<BehaviorTree>();
behaviourTree.SetVariableValue("waypoints", WayPointsManager.instance.GetWayPointsList());
}
}
On runtime I can see the waypoints are correctly added to the shared variable. But I still get the same error message from the beginning.
The error messages occures as soon a the task tries to access the waypoints...
C#:
public override void OnStart()
{
base.OnStart();
// initially move towards the closest waypoint
float distance = Mathf.Infinity;
float localDistance;
for (int i = 0; i < waypoints.Value.Count; ++i)
{
if ((localDistance = Vector3.Magnitude(transform.position - waypoints.Value[i].transform.position)) < distance) // here the error occures
{
distance = localDistance;
waypointIndex = i;
}
}
waypointReachedTime = -1;
SetDestination(Target());
}
When I add the waypoints manually from the scene to the behaviour tree everything works fine... but I need to add the waypoints on runtime, because the gameobject with the bt is a prefab
I would say no. I disabeld the "Start When Enabled" option on the bt. Then I added the waypoints and after that I enabled the bt and got the same outcome.Could it be a "race condition" and waypoints are assigned after the task has started?
C#:
void Start()
{
BehaviorTree behaviourTree = GetComponent<BehaviorTree>();
behaviourTree.SetVariableValue("waypoints", WayPointsManager.instance.GetWayPointsList());
behaviourTree.EnableBehavior();
}
UPDATE:
I just tried to use the original patrol task from the movement pack and changed assigned the waypoints variable to the shared variable. And then added the waypoints on runtime. I get the same error.
Last edited: