OhJeongSeok
New member
Hello.
I'm developing the zombie FPS game.
My zombie has Behaviour tree.
Each zombie trace a player and attack a player.
I have a problem.
My game spawn a zombie using pool system.
When zombie spawned, zombie object activated.
There is a lot of overhead in the Behaviour.Start() function when zombies are spawned.
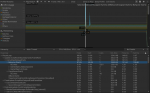
For this reason, the frame is dropped.
As I was looking for a solution, I saw how to allocate externalBehaviour using a pool.
As in my case, if each zombie is chasing and attacking the player, can I use the external behavior pool to get a performance advantage?
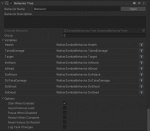
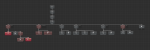
How to solve this problem?
Thank you for reading
I'm developing the zombie FPS game.
My zombie has Behaviour tree.
Each zombie trace a player and attack a player.
I have a problem.
My game spawn a zombie using pool system.
When zombie spawned, zombie object activated.
There is a lot of overhead in the Behaviour.Start() function when zombies are spawned.
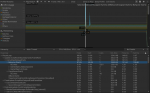
For this reason, the frame is dropped.
As I was looking for a solution, I saw how to allocate externalBehaviour using a pool.
As in my case, if each zombie is chasing and attacking the player, can I use the external behavior pool to get a performance advantage?
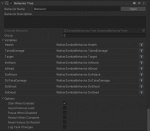
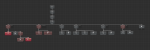
How to solve this problem?
Thank you for reading