black panther
Member
Hello, i have a question regarding the Damage system in opsive..
i want to make a headshot system, so i put a hitcollider in my player head and attach the hitcollider in player character health component on my character and set the multiplier value to 0, the goal is to catch if the player was hit in the head (compare by tag) and the damage is 0 i will apply damage to the character equal to its health value so the character will automatically dead..
but i encounter a strange behavior where its run very nice when i shoot the character from the short distance, but it will not work from long distance..
below i attach the setup, code, and video.
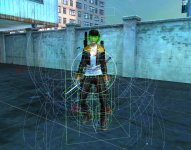
i want to make a headshot system, so i put a hitcollider in my player head and attach the hitcollider in player character health component on my character and set the multiplier value to 0, the goal is to catch if the player was hit in the head (compare by tag) and the damage is 0 i will apply damage to the character equal to its health value so the character will automatically dead..
but i encounter a strange behavior where its run very nice when i shoot the character from the short distance, but it will not work from long distance..
below i attach the setup, code, and video.
Code:
using Opsive.Shared.Events;
using Opsive.UltimateCharacterController.Traits;
using Opsive.UltimateCharacterController.Traits.Damage;
using UnityEngine;
public class HeadshootHandler : MonoBehaviour
{
private CharacterHealth _playerHealth;
public void Awake()
{
EventHandler.RegisterEvent<DamageData>(gameObject, "OnHealthDamageWithData", OnDamageWithData);
_playerHealth = this.GetComponent<CharacterHealth>();
}
//detect when the player is attacked
private void OnDamageWithData(DamageData damageData)
{
if (damageData.HitCollider == null)
return;
if (damageData.HitCollider.CompareTag("HeadCollider"))
{
DamageData headshootDamageData = new DamageData();
headshootDamageData.Amount = _playerHealth.Value;
headshootDamageData.Direction = Vector3.zero; //TIO: not sure what to put here
headshootDamageData.Position = transform.position; //TIO: not sure what to put here
headshootDamageData.Radius = 1; //TIO: not sure what to put here
headshootDamageData.Frames = 1; //TIO: not sure what to put here
headshootDamageData.ForceMagnitude = 0; //TIO: not sure what to put here
headshootDamageData.HitCollider = damageData.HitCollider; //TIO: this is from the parameter
headshootDamageData.DamageOriginator = damageData.DamageOriginator;
headshootDamageData.DamageTarget = GetComponent<CharacterHealth>();
//TIO: flag to tell that this is a headshot
headshootDamageData.UserData = "hs";
_playerHealth.Damage(headshootDamageData);
if (_playerHealth.IsAlive())
_playerHealth.ImmediateDeath();
}
else
{
Debug.Log("head collider not detected: " + damageData.HitCollider);
}
}
public void OnDestroy()
{
EventHandler.UnregisterEvent<DamageData>(gameObject, "OnHealthDamageWithData", OnDamageWithData);
}
}
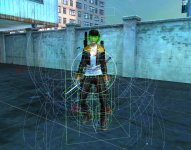