I have a custom script which allows me to open a drawer, however when I put a pickup item inside the drawer I can't open it anymore. The items is small enough to fit inside the drawer. The problem is when the sphere collider (trigger) of the pickup item collides with the drawer collider. But I can't make it smaller otherwise the player with not be able to pick up the item.
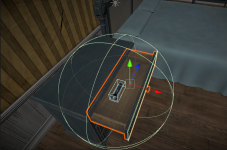
My custom script is this one:
Don't mind about the word "door". I just modified a door script to do the same action with the drawer.
This is my layer collision matrix if it helps:
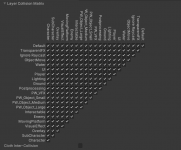
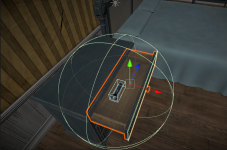
My custom script is this one:
C#:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class drawer : MonoBehaviour
{
[SerializeField] float ySensitivity = 300f;
[SerializeField] float frontOpenPosLimit = 45;
[SerializeField] GameObject frontDoorCollider;
bool moveDoor = false;
DoorCollision doorCollision = DoorCollision.NONE;
// Use this for initialization
void Start()
{
StartCoroutine(doorMover());
}
// Update is called once per frame
void Update()
{
if (Input.GetMouseButtonDown(0))
{
Debug.Log("Mouse down");
RaycastHit hitInfo = new RaycastHit();
if (Physics.Raycast(Camera.main.ScreenPointToRay(Input.mousePosition), out hitInfo))
{
if (hitInfo.collider.gameObject == frontDoorCollider)
{
moveDoor = true;
Debug.Log("Front door hit");
doorCollision = DoorCollision.FRONT;
}
else
{
doorCollision = DoorCollision.NONE;
}
}
}
if (Input.GetMouseButtonUp(0))
{
moveDoor = false;
Debug.Log("Mouse up");
}
}
IEnumerator doorMover()
{
bool stoppedBefore = false;
float zPos = 0;
while (true)
{
if (moveDoor)
{
stoppedBefore = false;
Debug.Log("Moving Drawer");
zPos += Input.GetAxis("Mouse Y") * ySensitivity * Time.deltaTime;
//Check if this is front door or back
if (doorCollision == DoorCollision.FRONT)
{
Debug.Log("Pull Down(PULL TOWARDS)");
zPos = Mathf.Clamp(zPos, -frontOpenPosLimit, 0);
Debug.Log(zPos);
transform.localPosition = new Vector3(0, 0, -zPos);
}
}
else
{
if (!stoppedBefore)
{
stoppedBefore = true;
Debug.Log("Stopped Moving Drawer");
}
}
yield return null;
}
}
enum DoorCollision
{
NONE, FRONT
}
}
Don't mind about the word "door". I just modified a door script to do the same action with the drawer.
This is my layer collision matrix if it helps:
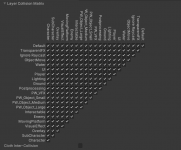