Xanto Nemot
New member
Hi Justin!
I don't know if this is strictly BD's domain or not, and I know it's potentially a niche use-case, but I figured I'd run it through you and see if you can tell me if this is something I can do myself or if it'd be a thing that could be added at some point.
Here's the deal. I'm using some enums with the attribute [System.Flags] on it, for example:
When I expose those as public vars on normal Monobehaviors, this is how the Inspector presents them:
gyazo.com
Meaning, I can select more than one at the same time, so I can use the single enum for flagging.
Now, I'd like to use that flag enum as well inside a Behavior, as a Shared variable, and so I added this class:
It works, the variable gets exposed and the code admits the use of it as an enum flag. However, inside any BD component, it only allows me to select one option at a time, which defeats the purpose of using enum flags:
gyazo.com
Furthermore, I've seen that you have successfully been able to implement the behavior I need with the SharedLayerMask class, so I wonder what I'm doing differently, and if I could achieve this the same way you did.
Thank you beforehand,
Xanto
I don't know if this is strictly BD's domain or not, and I know it's potentially a niche use-case, but I figured I'd run it through you and see if you can tell me if this is something I can do myself or if it'd be a thing that could be added at some point.
Here's the deal. I'm using some enums with the attribute [System.Flags] on it, for example:
C#:
[System.Serializable]
[System.Flags]
public enum InteractionType
{
None = 0,
All = ~0,
CanForceGrab = (1 << 0),
CanForceMove = (1 << 1),
CanForceThrow = (1 << 2),
IsForceGrabbing = (1 << 3),
IsForceMoving = (1 << 4),
IsForceThrowing = (1 << 5),
IsColliding = (1 << 6)
}
When I expose those as public vars on normal Monobehaviors, this is how the Inspector presents them:
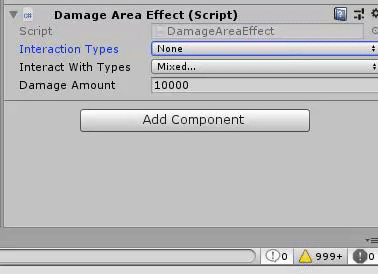
Gyazo Screen Video
Meaning, I can select more than one at the same time, so I can use the single enum for flagging.
Now, I'd like to use that flag enum as well inside a Behavior, as a Shared variable, and so I added this class:
C#:
using BehaviorDesigner.Runtime;
[System.Serializable]
public class SharedInteractionType : SharedVariable<InteractionType>
{
public static implicit operator SharedInteractionType(InteractionType value) { return new SharedInteractionType { Value = value }; }
}
It works, the variable gets exposed and the code admits the use of it as an enum flag. However, inside any BD component, it only allows me to select one option at a time, which defeats the purpose of using enum flags:
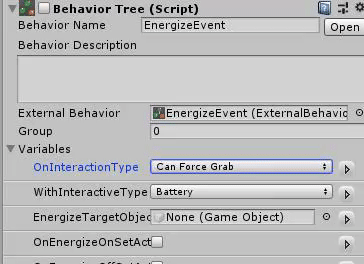
Gyazo Screen Video
Furthermore, I've seen that you have successfully been able to implement the behavior I need with the SharedLayerMask class, so I wonder what I'm doing differently, and if I could achieve this the same way you did.
Thank you beforehand,
Xanto
Last edited: